canvasに画像をタイル状で並べる[画像を繰り返し塗りつぶす]
HTML5のcanvasで画像をタイル状に並べる方法をご紹介します。自分で関数などを作成する必要はなく標準機能で可能です。
まずは実行結果から
[使用する画像]

[タイル状にならべた結果]
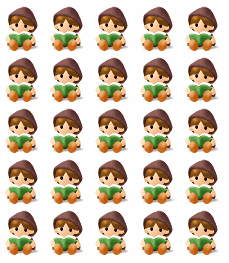
プログラムコード
<!DOCTYPE html> <html> <head> <meta charset="UTF-8"> </head> <body> <canvas id="MyCanvas"></canvas> <img id="img_test" src="test.gif" style="display:none;"> <script> // キャンバスの取得 var canvas = document.getElementById("MyCanvas"); if (canvas.getContext) { // コンテキストの取得 var ctx = canvas.getContext("2d"); // イメージの取得 var image = document.getElementById("img_test"); // イメージが読み込まれた image.addEventListener("load", function () { // 対象のイメージを5個分配置する canvas.width = image.width * 5; canvas.height = image.height * 5; // 現在のパスをリセットする ctx.beginPath(); // 繰り返しパターンの作成 var pattern = ctx.createPattern(image, 'repeat'); // 塗りつぶしスタイルの設定 ctx.fillStyle = pattern; // パスに矩形のサブパスを追加する ctx.rect(0,0,canvas.width,canvas.height); // 現在の塗りつぶしスタイルでサブパスを塗りつぶす ctx.fill(); },false); } </script> </body> </html>
繰り返しパターン
29行目のcontext.createPattern()の第一引数にはイメージ、第二引数には繰り返しパターンを設定します。この例では「repeat」を指定していますので垂直方向/水平方向の両方向に対して繰り返しをしています。
第二引数に「repeat-x」を指定すると水平方向のみ繰り返します。
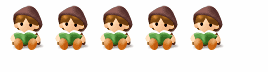
第二引数に「repeat-y」を指定すると垂直方向のみ繰り返します。
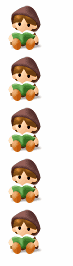
その他
タイル状に塗りつぶす対象はサブパスですので、円形/三角形なども可能です。
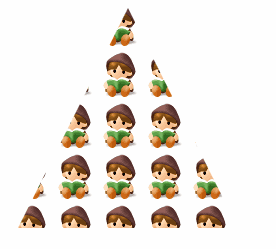
<!DOCTYPE html> <html> <head> <meta charset="UTF-8"> </head> <body> <canvas id="MyCanvas"></canvas> <img id="img_test" src="test.gif" style="display:none;"> <script> // キャンバスの取得 var canvas = document.getElementById("MyCanvas"); if (canvas.getContext) { // コンテキストの取得 var ctx = canvas.getContext("2d"); // イメージの取得 var image = document.getElementById("img_test"); // イメージが読み込まれた image.addEventListener("load", function () { canvas.width = 300; canvas.height = 300; // 現在のパスをリセットする ctx.beginPath(); // 繰り返しパターンの作成 var pattern = ctx.createPattern(image, 'repeat'); // 塗りつぶしスタイルの設定 ctx.fillStyle = pattern; // パスに三角形のサブパスを追加する ctx.moveTo(120, 10); ctx.lineTo(10, 230); ctx.lineTo(230, 230); ctx.closePath(); // 現在の塗りつぶしスタイルでサブパスを塗りつぶす ctx.fill(); },false); } </script> </body> </html>
以上となります。
スポンサーリンク
関連記事
公開日:2016年02月17日
記事NO:01771
この記事を書いた人
![]() | 💻 ITスキル・経験 サーバー構築からWebアプリケーション開発。IoTをはじめとする電子工作、ロボット、人工知能やスマホ/OSアプリまで分野問わず経験。 画像処理/音声処理/アニメーション、3Dゲーム、会計ソフト、PDF作成/編集、逆アセンブラ、EXE/DLLファイルの書き換えなどのアプリを公開。詳しくは自己紹介へ |
プチモンテ代表、アーティスト名:プチモンテ | |
🎵 音楽制作 BGMは楽器(音源)さえあれば、何でも制作可能。歌モノは主にロック、バラード、ポップスを制作。歌詞は叙情詩、叙情的な楽曲が多い。楽曲制作は2023年12月中旬 ~ |
オリジナル曲を始めました✨
YouTubeで各楽曲を公開しています🌈
https://www.youtube.com/@petitmonte