WindowsAPIの使い方 [ExcelのVBA]
Windows API
Windows APIのAPI(Application Programming Interface)とはVBAでいうと関数や手続きのようなプロシージャの総称です。32bitで動作するAPIはWin32APIとも呼ばれます。
APIの使い方
使い方としては「APIを宣言してAPIを使用する」という流れになります。
APIの使用方法や引数の解説などはマイクロソフトのMSDNのサイトにありますが、残念ながらAPIを宣言する宣言文が現在では公式からは入手困難となっています。
但し、ネットでの検索や私が作成した約100個の「APIサンプル集」などから宣言文は入手可能です。
※APIサンプル集はVisualBasic 5.0を対象としている為、VBAで使用する為には若干の修正が必要となります。
APIサンプル集の使用例
MIDI音源を使用してピアノの鍵盤を押すと「ドレミファソラシド」と音が鳴るサンプルをご紹介します。
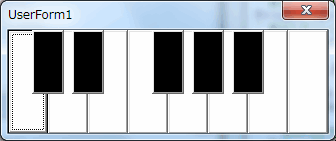
APIサンプル集の元のページは「MIDI音源を使用して音を鳴らす」です。
前述のページでvbapi_midi.zipをダウンロードして展開すると「MIDI.frm」というファイルがあります。このファイルを秀丸エディタのような高機能なエディタで開きます。
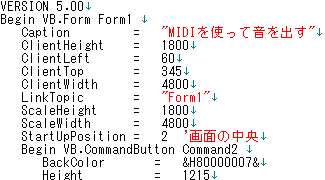
すると、上部にフォームやコマンドボタンなどの記述がありプログラムのコードはその後に続きます。

VBAではこのファイルは開けませんのでプログラムのコードの部分をコピペして流用します。※コピペしてもそのまま使えない場合があります。その場合はVBA用にコードを変更します。
VBA用に変換したコード
Option Explicit ' *** APIの宣言 *** ' MIDI出力デバイス数を取得する Private Declare Function midiOutGetNumDevs Lib "winmm" () As Integer ' MIDIデバイスを開く Private Declare Function midiOutOpen Lib "winmm.dll" (lphMidiOut As Long, ByVal uDeviceID As Long, ByVal dwCallback As Long, ByVal dwInstance As Long, ByVal dwFlags As Long) As Long ' MIDIデバイスから音をだす Private Declare Function midiOutShortMsg Lib "winmm.dll" (ByVal hMidiOut As Long, ByVal dwMsg As Long) As Long ' MIDIデバイスを閉じる Private Declare Function midiOutClose Lib "winmm.dll" (ByVal hMidiOut As Long) As Long Dim Handle As Long ' MIDIデバイスのハンドル Private Sub UserForm_Initialize() Dim Ret As Long ' MIDI出力デバイス数を取得する Ret = midiOutGetNumDevs If Ret = 0 Then MsgBox "MIDI音源がないのでこのサンプルはご利用できません。" Else ' MIDIデバイスを開く Ret = midiOutOpen(Handle, -1, 0, 0, 0) End If ' ※MIDIデバイスを開いたら終了時には必ず閉じてください。 ' ※音がならなくなった場合は一旦、エクセルを終了させてください。 CommandButton1.Tag = 1 CommandButton2.Tag = 2 CommandButton3.Tag = 3 CommandButton4.Tag = 4 CommandButton5.Tag = 5 CommandButton6.Tag = 6 CommandButton7.Tag = 7 CommandButton8.Tag = 8 CommandButton9.Tag = 9 CommandButton10.Tag = 10 CommandButton11.Tag = 11 CommandButton12.Tag = 12 CommandButton13.Tag = 13 End Sub Private Sub UserForm_Terminate() Dim Ret As Long ' MIDIデバイスを閉じる Ret = midiOutClose(Handle) End Sub Private Sub MouseDown(Tag As Long) Dim Msg As Long ' 音階 Select Case Tag Case 1 'ド Msg = &H7F3C90 Call midiOutShortMsg(Handle, Msg) Case 2 'レ Msg = &H7F3C90 + 2 * 256 Call midiOutShortMsg(Handle, Msg) Case 3 'ミ Msg = &H7F3C90 + 4 * 256 Call midiOutShortMsg(Handle, Msg) Case 4 'ファ Msg = &H7F3C90 + 5 * 256 Call midiOutShortMsg(Handle, Msg) Case 5 'ソ Msg = &H7F3C90 + 7 * 256 Call midiOutShortMsg(Handle, Msg) Case 6 'ラ Msg = &H7F3C90 + 9 * 256 Call midiOutShortMsg(Handle, Msg) Case 7 'シ Msg = &H7F3C90 + 11 * 256 Call midiOutShortMsg(Handle, Msg) Case 8 'ド Msg = &H7F3C90 + 12 * 256 Call midiOutShortMsg(Handle, Msg) Case 9 'ド# Msg = &H7F3C90 + 1 * 256 Call midiOutShortMsg(Handle, Msg) Case 10 'レ# Msg = &H7F3C90 + 3 * 256 Call midiOutShortMsg(Handle, Msg) Case 11 'ファ# Msg = &H7F3C90 + 6 * 256 Call midiOutShortMsg(Handle, Msg) Case 12 'ソ# Msg = &H7F3C90 + 8 * 256 Call midiOutShortMsg(Handle, Msg) Case 13 'ラ# Msg = &H7F3C90 + 10 * 256 Call midiOutShortMsg(Handle, Msg) End Select End Sub Private Sub MouseUp(Tag As Long) Dim Msg As Long ' 音階 Select Case Tag Case 1 Msg = &H3C90 Call midiOutShortMsg(Handle, Msg) Case 2 Msg = &H3C90 + 2 * 256 Call midiOutShortMsg(Handle, Msg) Case 3 Msg = &H3C90 + 4 * 256 Call midiOutShortMsg(Handle, Msg) Case 4 Msg = &H3C90 + 5 * 256 Call midiOutShortMsg(Handle, Msg) Case 5 Msg = &H3C90 + 7 * 256 Call midiOutShortMsg(Handle, Msg) Case 6 Msg = &H3C90 + 9 * 256 Call midiOutShortMsg(Handle, Msg) Case 7 Msg = &H3C90 + 11 * 256 Call midiOutShortMsg(Handle, Msg) Case 8 Msg = &H3C90 + 12 * 256 Call midiOutShortMsg(Handle, Msg) Case 9 Msg = &H3C90 + 1 * 256 Call midiOutShortMsg(Handle, Msg) Case 10 Msg = &H3C90 + 3 * 256 Call midiOutShortMsg(Handle, Msg) Case 11 Msg = &H3C90 + 6 * 256 Call midiOutShortMsg(Handle, Msg) Case 12 Msg = &H3C90 + 8 * 256 Call midiOutShortMsg(Handle, Msg) Case 13 Msg = &H3C90 + 10 * 256 Call midiOutShortMsg(Handle, Msg) End Select End Sub ' 以下は長文になっていますが、単純なコードにまとめる事が出来ると思います。 Private Sub CommandButton1_MouseDown(ByVal Button As Integer, ByVal Shift As Integer, ByVal X As Single, ByVal Y As Single) MouseDown (CommandButton1.Tag) End Sub Private Sub CommandButton1_MouseUp(ByVal Button As Integer, ByVal Shift As Integer, ByVal X As Single, ByVal Y As Single) MouseUp (CommandButton1.Tag) End Sub Private Sub CommandButton2_MouseDown(ByVal Button As Integer, ByVal Shift As Integer, ByVal X As Single, ByVal Y As Single) MouseDown (CommandButton2.Tag) End Sub Private Sub CommandButton2_MouseUp(ByVal Button As Integer, ByVal Shift As Integer, ByVal X As Single, ByVal Y As Single) MouseUp (CommandButton2.Tag) End Sub Private Sub CommandButton3_MouseDown(ByVal Button As Integer, ByVal Shift As Integer, ByVal X As Single, ByVal Y As Single) MouseDown (CommandButton3.Tag) End Sub Private Sub CommandButton3_MouseUp(ByVal Button As Integer, ByVal Shift As Integer, ByVal X As Single, ByVal Y As Single) MouseUp (CommandButton3.Tag) End Sub Private Sub CommandButton4_MouseDown(ByVal Button As Integer, ByVal Shift As Integer, ByVal X As Single, ByVal Y As Single) MouseDown (CommandButton4.Tag) End Sub Private Sub CommandButton4_MouseUp(ByVal Button As Integer, ByVal Shift As Integer, ByVal X As Single, ByVal Y As Single) MouseUp (CommandButton4.Tag) End Sub Private Sub CommandButton5_MouseDown(ByVal Button As Integer, ByVal Shift As Integer, ByVal X As Single, ByVal Y As Single) MouseDown (CommandButton5.Tag) End Sub Private Sub CommandButton5_MouseUp(ByVal Button As Integer, ByVal Shift As Integer, ByVal X As Single, ByVal Y As Single) MouseUp (CommandButton5.Tag) End Sub Private Sub CommandButton6_MouseDown(ByVal Button As Integer, ByVal Shift As Integer, ByVal X As Single, ByVal Y As Single) MouseDown (CommandButton6.Tag) End Sub Private Sub CommandButton6_MouseUp(ByVal Button As Integer, ByVal Shift As Integer, ByVal X As Single, ByVal Y As Single) MouseUp (CommandButton6.Tag) End Sub Private Sub CommandButton7_MouseDown(ByVal Button As Integer, ByVal Shift As Integer, ByVal X As Single, ByVal Y As Single) MouseDown (CommandButton7.Tag) End Sub Private Sub CommandButton7_MouseUp(ByVal Button As Integer, ByVal Shift As Integer, ByVal X As Single, ByVal Y As Single) MouseUp (CommandButton7.Tag) End Sub Private Sub CommandButton8_MouseDown(ByVal Button As Integer, ByVal Shift As Integer, ByVal X As Single, ByVal Y As Single) MouseDown (CommandButton8.Tag) End Sub Private Sub CommandButton8_MouseUp(ByVal Button As Integer, ByVal Shift As Integer, ByVal X As Single, ByVal Y As Single) MouseUp (CommandButton8.Tag) End Sub Private Sub CommandButton9_MouseDown(ByVal Button As Integer, ByVal Shift As Integer, ByVal X As Single, ByVal Y As Single) MouseDown (CommandButton9.Tag) End Sub Private Sub CommandButton9_MouseUp(ByVal Button As Integer, ByVal Shift As Integer, ByVal X As Single, ByVal Y As Single) MouseUp (CommandButton9.Tag) End Sub Private Sub CommandButton10_MouseDown(ByVal Button As Integer, ByVal Shift As Integer, ByVal X As Single, ByVal Y As Single) MouseDown (CommandButton10.Tag) End Sub Private Sub CommandButton10_MouseUp(ByVal Button As Integer, ByVal Shift As Integer, ByVal X As Single, ByVal Y As Single) MouseUp (CommandButton10.Tag) End Sub Private Sub CommandButton11_MouseDown(ByVal Button As Integer, ByVal Shift As Integer, ByVal X As Single, ByVal Y As Single) MouseDown (CommandButton11.Tag) End Sub Private Sub CommandButton11_MouseUp(ByVal Button As Integer, ByVal Shift As Integer, ByVal X As Single, ByVal Y As Single) MouseUp (CommandButton11.Tag) End Sub Private Sub CommandButton12_MouseDown(ByVal Button As Integer, ByVal Shift As Integer, ByVal X As Single, ByVal Y As Single) MouseDown (CommandButton12.Tag) End Sub Private Sub CommandButton12_MouseUp(ByVal Button As Integer, ByVal Shift As Integer, ByVal X As Single, ByVal Y As Single) MouseUp (CommandButton12.Tag) End Sub Private Sub CommandButton13_MouseDown(ByVal Button As Integer, ByVal Shift As Integer, ByVal X As Single, ByVal Y As Single) MouseDown (CommandButton13.Tag) End Sub Private Sub CommandButton13_MouseUp(ByVal Button As Integer, ByVal Shift As Integer, ByVal X As Single, ByVal Y As Single) MouseUp (CommandButton13.Tag) End Sub
このコードとフォームが設定してあるファイルをダウンロードする事が出来ます。但し、マクロ有効ブック(*.xlsm)となりますので、不安な方は上記のコードからフォームをご自分で設定して下さい。
excel_vba_62_1.xlsm 22.2 KB (22,740 バイト)
[VBAのフォーム]
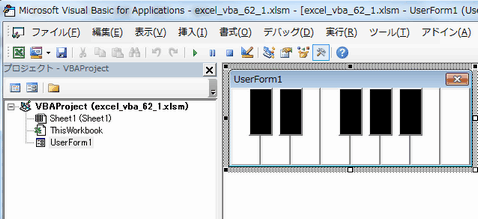
以上でAPIの使い方は終了となります。基本的に「APIサンプル集」のコードを試行錯誤しながら使うとAPIは覚えられると思います。