PNG/JPEG/GIFファイルをPNG/JPEGファイルへ変換する[HTML5の標準機能]
ブラウザでPNG/JPEG/GIFファイルを読み込んでPNGまたはJPEGファイルに変換するサンプルです。これらの変換はHTML5の標準機能でJavascriptのみで変換する事ができます。
変換画面
ファイルを変換する実行画面です。
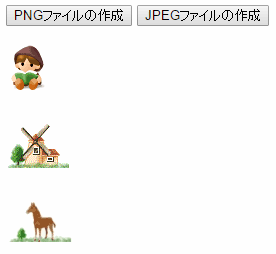
[作成したPNGファイル]
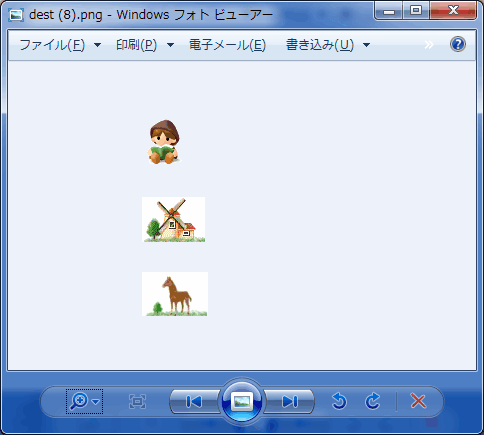
[作成したJPEGファイル]
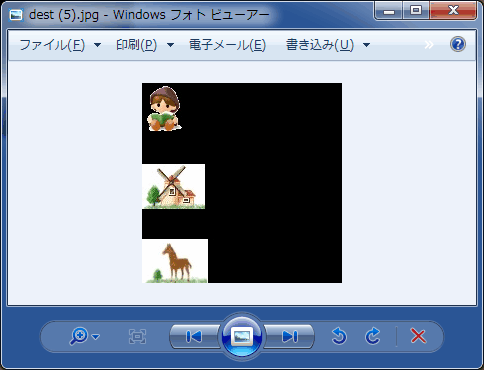
PNGファイルはアルファチャンネルがある為、背景は透明になっていますが、JPEGファイルはアルファチャンネルがありませんのでデフォルトの背景色である「黒色」となります。背景色はcanvasで設定可能です。
背景色を白色に変更するには72行目あたりに次のコードを追加します。
ctx.fillStyle = '#ffffff';
ctx.fillRect(0,0, canvas.width,canvas.height);
ctx.fillRect(0,0, canvas.width,canvas.height);
動作確認
IE11 / Edge / Chrome / FireFox
※IE9/IE10は未確認ですが動作するかも知れません。
変換するプログラム
プログラムの流れとしてはPNG/JPEG/GIFファイルを読み込んでcanvasへ描画します。そして、ボタンを押した時にPNGファイルまたはJPEGファイルを作成します。
<!DOCTYPE html> <html> <head> <meta charset="UTF-8"> <script> function AsciiToUint8Array (S) { var len = S.length; var P = new Uint8Array(len); for (var i = 0; i < len; i++) { P[i] = S[i].charCodeAt(0); } return P; } function SaveToFile(Stream,FileName) { // IE/Edge if (window.navigator.msSaveBlob) { window.navigator.msSaveBlob(new Blob([Stream]), FileName); // それ以外 } else { var a = document.createElement("a"); a.href = URL.createObjectURL(new Blob([Stream])); //a.target = '_blank'; a.download = FileName; document.body.appendChild(a) // FF specification a.click(); document.body.removeChild(a) // FF specification } } function onClick(option){ // PNGファイルの作成 if (option == 1){ var png = canvas.toDataURL("image/png").replace("data:image/png;base64,",""); png = window.atob(png); var Stream = AsciiToUint8Array(png); SaveToFile(Stream,'dest.png'); } // JEPGファイルの作成 if (option == 2){ var png = canvas.toDataURL("image/jpeg").replace("data:image/jpeg;base64,",""); png = window.atob(png); var Stream = AsciiToUint8Array(png); SaveToFile(Stream,'dest.jpg'); } } </script> </head> <body> <img id="img_gif" src="test.gif" style = "display:none;"> <img id="img_png" src="test.png" style = "display:none;"> <img id="img_jpg" src="test.jpg" style = "display:none;"> <button onclick="onClick(1);">PNGファイルの作成</button> <button onclick="onClick(2);">JPEGファイルの作成</button> <br><br> <canvas id="MyCanvas"></canvas> <script> // キャンバスの取得 var canvas = document.getElementById("MyCanvas"); canvas.width = 200; canvas.height = 200; // コンテキストの取得 var ctx = canvas.getContext("2d"); var max_height = 0; var imglist = new Array(); imglist[0] = document.getElementById("img_gif"); imglist[1] = document.getElementById("img_png"); imglist[2] = document.getElementById("img_jpg"); // キャンバスに画像を描画 imglist[0].onload = function() { ctx.drawImage(imglist[0],0,max_height); max_height += imglist[0].height+30; }; // キャンバスに画像を描画 imglist[1].onload = function() { ctx.drawImage(imglist[1],0,max_height); max_height += imglist[1].height+30; }; // キャンバスに画像を描画 imglist[2].onload = function() { ctx.drawImage(imglist[2],0,max_height); max_height += imglist[2].height+30; }; </script> </body> </html>
変換処理の部分はcanvas.toDataURL()でキャンバスの内容をBasae64形式のデータファイル(文字列)に変換します。その後にファイル固有のヘッダを削除します。
そして、残りのBase64の文字列をバイナリデータに変換してファイルをダウンロードさせる流れとなります。
スポンサーリンク
関連記事
公開日:2016年02月20日
記事NO:01783