バックグラウンド処理(サービス) [Android Studio]
目次
1. 作るもの
2. 画面設計
2-1. メイン
2-2. サービス
3. コーディング
1. 作るもの
再生ボタンを押すとサービスを開始します。サービスはMediaPlayerを使用して通知音を繰り返し再生します。停止ボタンを押すとサービスを停止して再生も終了します。
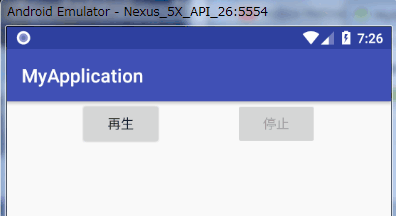
2. 画面設計
2-1. メイン
Buttonを2個配置します。
<?xml version="1.0" encoding="utf-8"?> <android.support.constraint.ConstraintLayout xmlns:android="http://schemas.android.com/apk/res/android" xmlns:app="http://schemas.android.com/apk/res-auto" xmlns:tools="http://schemas.android.com/tools" android:layout_width="match_parent" android:layout_height="match_parent" tools:context=".MainActivity"> <Button android:id="@+id/button" android:layout_width="wrap_content" android:layout_height="wrap_content" android:text="再生" app:layout_constraintEnd_toStartOf="@+id/button2" app:layout_constraintHorizontal_bias="0.5" app:layout_constraintStart_toStartOf="parent" app:layout_constraintTop_toTopOf="parent" /> <Button android:id="@+id/button2" android:layout_width="wrap_content" android:layout_height="wrap_content" android:text="停止" app:layout_constraintBottom_toBottomOf="@+id/button" app:layout_constraintEnd_toEndOf="parent" app:layout_constraintHorizontal_bias="0.5" app:layout_constraintStart_toEndOf="@+id/button" app:layout_constraintTop_toTopOf="@+id/button" /> </android.support.constraint.ConstraintLayout>
2-2. サービス
[新規][サービス]で「サービス」を選択します。
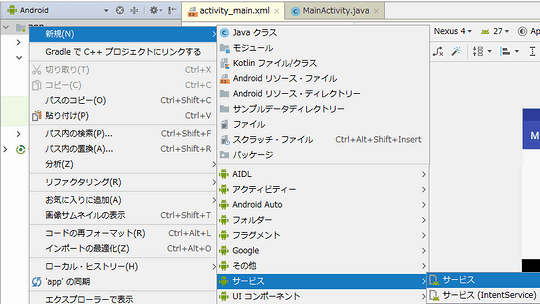
クラス名を「MyService」でエキスポート済みを「オフ」にする。
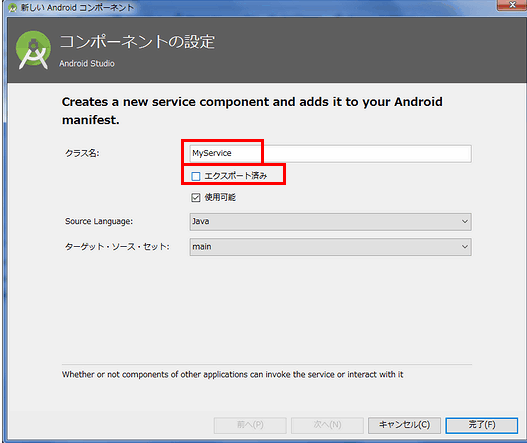
サービスをプロジェクトに追加するとapp\manifests\AndroidManifest.xmlに次のコードが自動で追記されます。
<service android:name=".MyService" android:enabled="true" android:exported="false"></service>
これで画面設計は完了です。次はコーディングです。
3. コーディング
[MainActivity.java]
import android.content.Intent; import android.support.v7.app.AppCompatActivity; import android.os.Bundle; import android.view.View; public class MainActivity extends AppCompatActivity { @Override protected void onCreate(Bundle savedInstanceState) { super.onCreate(savedInstanceState); setContentView(R.layout.activity_main); // ボタンの制御 findViewById(R.id.button).setEnabled(true); findViewById(R.id.button2).setEnabled(false); findViewById(R.id.button).setOnClickListener(new View.OnClickListener() { @Override public void onClick(View v) { // サービスの開始 Intent intent = new Intent(MainActivity.this,MyService.class); startService(intent); findViewById(R.id.button).setEnabled(false); findViewById(R.id.button2).setEnabled(true); } }); findViewById(R.id.button2).setOnClickListener(new View.OnClickListener() { @Override public void onClick(View v) { Intent intent = new Intent(MainActivity.this,MyService.class); stopService(intent); findViewById(R.id.button).setEnabled(true); findViewById(R.id.button2).setEnabled(false); } }); } }
[MyService.java]
import android.app.Service; import android.content.Intent; import android.media.AudioManager; import android.media.MediaPlayer; import android.media.RingtoneManager; import android.net.Uri; import android.os.IBinder; public class MyService extends Service { private MediaPlayer player; @Override public void onCreate() { player = new MediaPlayer(); } @Override public int onStartCommand(Intent intent, int flags, int startId) { // メディアの音量調整シークバーを表示する // ※今回は初期値を最大音量にしています。 AudioManager manager = (AudioManager)getSystemService(MyService.this.AUDIO_SERVICE);; manager.setStreamVolume(AudioManager.STREAM_MUSIC, manager.getStreamMaxVolume(AudioManager.STREAM_MUSIC), AudioManager.FLAG_SHOW_UI); // 通知音 Uri uri = RingtoneManager.getDefaultUri(RingtoneManager.TYPE_NOTIFICATION); try { // 通知音を繰り返し再生する player.setDataSource(MyService.this, uri); player.setLooping(true); player.prepare(); player.start(); }catch (Exception e){ e.getStackTrace(); } // START_NOT_STICKY // サービスが終了されてもサービスを再起動しない(常にサービスを起動しない) // ※ココの設定次第で常にサービスを起動する事が可能です。 return START_NOT_STICKY; } @Override public void onDestroy() { if(player.isPlaying()) { player.stop(); } player.release(); player = null; } @Override public IBinder onBind(Intent intent) { // TODO: Return the communication channel to the service. throw new UnsupportedOperationException("Not yet implemented"); } }
参考URL(Google)
スポンサーリンク
関連記事
前の記事: | SQLiteによるデータベース操作(SELECT/INSERT/UPDATE/DELETE/トランザクション) [Android Studio] |
次の記事: | 通知エリアに「通知」(Notification)を表示する [Android Studio] |
公開日:2018年05月22日
記事NO:02662
この記事を書いた人
![]() | 💻 ITスキル・経験 サーバー構築からWebアプリケーション開発。IoTをはじめとする電子工作、ロボット、人工知能やスマホ/OSアプリまで分野問わず経験。 画像処理/音声処理/アニメーション、3Dゲーム、会計ソフト、PDF作成/編集、逆アセンブラ、EXE/DLLファイルの書き換えなどのアプリを公開。詳しくは自己紹介へ |
プチモンテ代表、アーティスト名:プチモンテ | |
🎵 音楽制作 BGMは楽器(音源)さえあれば、何でも制作可能。歌モノは主にロック、バラード、ポップスを制作。歌詞は叙情詩、叙情的な楽曲が多い。楽曲制作は2023年12月中旬 ~ |
オリジナル曲を始めました✨
YouTubeで各楽曲を公開しています🌈
https://www.youtube.com/@petitmonte