Rails6にVue.jsを導入する
1-a. 新規プロジェクトにVue.jsを追加する
新規プロジェクトにVue.jsを追加する場合は「--webpack=vue」とします。次の例ではTurbolinksなど不要な機能はスキップしています。
cd ~/ mkdir myapp cd myapp rails new . --webpack=vue --skip-turbolinks --skip-action-mailer --skip-action-mailbox --skip-active-storage --skip-test -d mysql
実行後、次のファイルが作成される。
app/javascript/app.vue |
app/javascript/packs/hello_vue.js |
[app/javascript/app.vue]
<template> <div id="app"> <p>{{ message }}</p> </div> </template> <script> export default { data: function () { return { message: "Hello Vue!" } } } </script> <style scoped> p { font-size: 2em; text-align: center; } </style>
※コードに関しては「8章 各コードの内容」で簡単に解説します。
[hello_vue.js]
/* eslint no-console: 0 */ // Run this example by adding <%= javascript_pack_tag 'hello_vue' %> (and // <%= stylesheet_pack_tag 'hello_vue' %> if you have styles in your component) // to the head of your layout file, // like app/views/layouts/application.html.erb. // All it does is render <div>Hello Vue</div> at the bottom of the page. import Vue from 'vue' import App from '../app.vue' document.addEventListener('DOMContentLoaded', () => { const app = new Vue({ render: h => h(App) }).$mount() document.body.appendChild(app.$el) console.log(app) }) // The above code uses Vue without the compiler, which means you cannot // use Vue to target elements in your existing html templates. You would // need to always use single file components. // To be able to target elements in your existing html/erb templates, // comment out the above code and uncomment the below // Add <%= javascript_pack_tag 'hello_vue' %> to your layout // Then add this markup to your html template: // // <div id='hello'> // {{message}} // <app></app> // </div> // import Vue from 'vue/dist/vue.esm' // import App from '../app.vue' // // document.addEventListener('DOMContentLoaded', () => { // const app = new Vue({ // el: '#hello', // data: { // message: "Can you say hello?" // }, // components: { App } // }) // }) // // // // If the project is using turbolinks, install 'vue-turbolinks': // // yarn add vue-turbolinks // // Then uncomment the code block below: // // import TurbolinksAdapter from 'vue-turbolinks' // import Vue from 'vue/dist/vue.esm' // import App from '../app.vue' // // Vue.use(TurbolinksAdapter) // // document.addEventListener('turbolinks:load', () => { // const app = new Vue({ // el: '#hello', // data: () => { // return { // message: "Can you say hello?" // } // }, // components: { App } // }) // })
さらに、次のファイルが編集される。※2行目から6行目まで追記される。
config/webpack/environment.js |
const { environment } = require('@rails/webpacker') const { VueLoaderPlugin } = require('vue-loader') const vue = require('./loaders/vue') environment.plugins.prepend('VueLoaderPlugin', new VueLoaderPlugin()) environment.loaders.prepend('vue', vue) module.exports = environment
1-b. 既存プロジェクトにVue.jsを追加する
rails webpacker:install:vue
※作成されるファイル、編集されるファイルに関しては1-aと同様。
2. コントローラー/ビューの作成
rails g controller Cat index
[app/views/cat/index.html.erb]
<h1>Cat#index</h1> <p>Find me in app/views/cat/index.html.erb</p>
3. application.html.erb
[app/views/layouts/application.html.erb]
<!DOCTYPE html> <html> <head> <title>Mpapp</title> <%= csrf_meta_tags %> <%= csp_meta_tag %> <%= stylesheet_link_tag 'application', media: 'all' %> <%= javascript_pack_tag 'application' %> <%# 以下の行を追加する。%> <%= javascript_pack_tag 'hello_vue' %> </head> <body> <%= yield %> </body> </html>
4. ルーティング
[config/routes.rb]
Rails.application.routes.draw do root to: 'cat#index' get 'cat/index' end
5. データベース
[config/database.yml]
development: <<: *default database: ココに各自の設定を記述 username: ココに各自の設定を記述 password: ココに各自の設定を記述
6. 実行する
bin/rails s
[実行例]
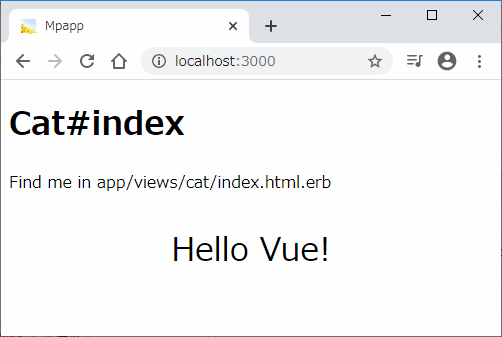
7. Vue.jsの使い方
日本語の公式サイトで解説されています。参考書なしでもいけます。
8. 各コードの内容
app.vue及びhello_vue.jsのコードの中身を確認すると「あれ?公式サイトにある基本と異なる・・・。」と思った方が多いでしょう。
公式サイトの「基本的な使い方」では次の「Hello Vue!」及び「コンポーネント」などを解説しています。
[Hello Vue!] ※公式サイトより抜粋
<div id="app"> {{ message }} </div> <script> var app = new Vue({ el: '#app', data: { message: 'Hello Vue!' } }) </script>
[コンポーネント] ※公式サイトより抜粋
<div id="components-demo"> <button-counter></button-counter> </div> <script> Vue.component('button-counter', { data: function () { return { count: 0 } }, template: '<button v-on:click="count++">You clicked me {{ count }} times.</button>' }) new Vue({ el: '#components-demo' }) </script>
このようなVue.jsの使い方は「小規模プロジェクト用」です。中規模以上の複雑なシステムでは.vueファイルを使用して開発を行います。
.vueファイルとは「単一ファイルコンポーネント」(VueComponent)と呼ばれていて、1つのファイルでテンプレート(HTML)、コンポーネント(JS)、CSSを定義します。
vueファイルに関してはVue Component の仕様(公式)、単一ファイルコンポーネント(公式)、スコープ付き CSS(公式)を参照。JSファイルの方はVue.jsのrender: h => h(App)について調べた(Qiita)をご覧ください。
その他に公式のAPIの仕様も確認すると良いでしょう。
関連記事
前の記事: | Uncaught TypeError: $(...).toast is not a function [Rails/bootstrap] |
次の記事: | Vue.jsをIE11に対応させる [Rails6] |
この記事を書いた人
![]() | 💻 ITスキル・経験 サーバー構築からWebアプリケーション開発。IoTをはじめとする電子工作、ロボット、人工知能やスマホ/OSアプリまで分野問わず経験。 画像処理/音声処理/アニメーション、3Dゲーム、会計ソフト、PDF作成/編集、逆アセンブラ、EXE/DLLファイルの書き換えなどのアプリを公開。詳しくは自己紹介へ |
プチモンテ代表、アーティスト名:プチモンテ | |
🎵 音楽制作 BGMは楽器(音源)さえあれば、何でも制作可能。歌モノは主にロック、バラード、ポップスを制作。歌詞は叙情詩、叙情的な楽曲が多い。楽曲制作は2023年12月中旬 ~ |
オリジナル曲を始めました✨
YouTubeで各楽曲を公開しています🌈
https://www.youtube.com/@petitmonte