Javaでテキスト/プロパティファイルの読み書きをする
目次
1. テキストファイル(UTF8)の書き込み
2. テキストファイル(UTF8)の読み込み
3. プロパティファイルの書き込み
4. プロパティファイルの読み込み
今回の例では「Javaプロジェクトの作業フォルダ」に各ファイルを作成します。最初の記事から進めている方は「C:\Pleiades\workspace\プロジェクト名」に各ファイルが作成されます。
1. テキストファイル(UTF8)の書き込み
import java.io.FileWriter; public class Main { public static void main(final String[] args){ FileWriter file = null; try { file = new FileWriter("テキストファイル.txt"); file.write("プチモンテ\r\n"); file.write("https://www.petitmonte.com/"); file.flush(); }catch (Exception e) { System.out.println(e.getMessage()); }finally { if(file != null) { try { file.close(); }catch(Exception e) { System.out.println(e.getMessage()); } } } } }
2. テキストファイル(UTF8)の読み込み
import java.io.FileReader; public class Main { public static void main(final String[] args){ FileReader file = null; try { file = new FileReader("テキストファイル.txt"); while(true) { // ファイルから一文字読み込む int i = file.read(); if( i == -1) { break; } System.out.print((char)i); } }catch (Exception e) { System.out.println(e.getMessage()); }finally { if(file != null) { try { file.close(); }catch(Exception e) { System.out.println(e.getMessage()); } } } } }
結果
プチモンテ
https://www.petitmonte.com/
https://www.petitmonte.com/
3. プロパティファイルの書き込み
プロパティファイルはWindowsのINIファイルと同様です。
import java.io.FileWriter; import java.util.Properties; public class Main { public static void main(final String[] args){ FileWriter file = null; try { file = new FileWriter("プロパティファイル.properties"); Properties p = new Properties(); p.setProperty("イギリス革命", "1641年"); p.setProperty("フランス革命", "1789年"); p.store(file, "history"); }catch (Exception e) { System.out.println(e.getMessage()); }finally { if(file != null) { try { file.close(); }catch(Exception e) { System.out.println(e.getMessage()); } } } } }
作成したファイルはメモ帳などで確認可能です。
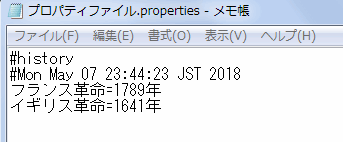
日付は自動的に挿入されます。また、historyの部分は日本語などのマルチバイト文字は使用できないようです。
4. プロパティファイルの読み込み
import java.io.FileReader; import java.util.Properties; public class Main { public static void main(final String[] args){ FileReader file = null; try { file = new FileReader("プロパティファイル.properties"); Properties p = new Properties(); p.load(file); System.out.println("イギリス革命:" + p.getProperty("イギリス革命")); System.out.println("フランス革命:" + p.getProperty("フランス革命")); }catch (Exception e) { System.out.println(e.getMessage()); }finally { if(file != null) { try { file.close(); }catch(Exception e) { System.out.println(e.getMessage()); } } } } }
結果
イギリス革命:1641年
フランス革命:1789年
フランス革命:1789年
スポンサーリンク
関連記事
公開日:2018年05月07日
記事NO:02647
この記事を書いた人
![]() | 💻 ITスキル・経験 サーバー構築からWebアプリケーション開発。IoTをはじめとする電子工作、ロボット、人工知能やスマホ/OSアプリまで分野問わず経験。 画像処理/音声処理/アニメーション、3Dゲーム、会計ソフト、PDF作成/編集、逆アセンブラ、EXE/DLLファイルの書き換えなどのアプリを公開。詳しくは自己紹介へ |
プチモンテ代表、アーティスト名:プチモンテ | |
🎵 音楽制作 BGMは楽器(音源)さえあれば、何でも制作可能。歌モノは主にロック、バラード、ポップスを制作。歌詞は叙情詩、叙情的な楽曲が多い。楽曲制作は2023年12月中旬 ~ |
オリジナル曲を始めました✨
YouTubeで各楽曲を公開しています🌈
https://www.youtube.com/@petitmonte